当我上高中的时候,我接触到的最早的编程语言之一就是 Logo。 它是交互式的,可视化的。 使用基本的移动命令,您可以让您的光标(“乌龟”)绘制基本的形状和复杂的图案。 这是一种介绍算法这个引人注目的概念的好方法——计算机执行的一系列指令。
幸运的是,Logo 编程语言现在可以作为 Python 包使用。 让我们直接开始,您可以随着我们的进行发现 Logo 的可能性。
安装 Turtle 模块
Logo 可以作为 Python 的 turtle
包 使用。 要使用它,您必须首先安装 Python。 Linux 和 BSD 上已经安装了 Python,并且在 MacOS 和 Windows 上都很容易安装。
安装 Python 后,安装 Turtle 模块
pip3 install turtle
Bob 画一个正方形
安装了 turtle
包后,您可以绘制一些基本形状。
要画一个正方形,想象一只乌龟(叫他 Bob)在您的屏幕中央,用尾巴拿着一支笔。 每次 Bob 移动,他都会在他身后画一条线。 Bob 必须如何移动才能画出一个正方形?
- 向前移动 100 步。
- 向右转 90 度。
- 向前移动 100 步。
- 向右转 90 度。
- 向前移动 100 步。
- 向右转 90 度。
- 向前移动 100 步。
现在用 Python 编写以上算法。 创建一个名为 logo.py
的文件并将以下代码放入其中。
import turtle
if __name__ == '__main__':
turtle.title('Hi! I\'m Bob the turtle!')
turtle.setup(width=800, height=800)
bob = turtle.Turtle(shape='turtle')
bob.color('orange')
# Drawing a square
bob.forward(100)
bob.right(90)
bob.forward(100)
bob.right(90)
bob.forward(100)
bob.right(90)
bob.forward(100)
turtle.exitonclick()
将以上代码另存为 logo.py
并运行它
$ python3 logo.py
Bob 在屏幕上画一个正方形
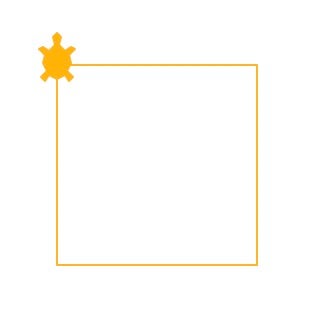
Ayush Sharma 插图,CC BY-SA 4.0
Bob 画一个六边形
要画一个六边形,Bob 必须这样移动
- 向前移动 150 步。
- 向右转 60 度。
- 向前移动 150 步。
- 向右转 60 度。
- 向前移动 150 步。
- 向右转 60 度。
- 向前移动 150 步。
- 向右转 60 度。
- 向前移动 150 步。
- 向右转 60 度。
- 向前移动 150 步。
在 Python 中,您可以使用 for
循环 来移动 Bob
import turtle
if __name__ == '__main__':
turtle.title('Hi! I\'m Bob the turtle!')
turtle.setup(width=800, height=800)
bob = turtle.Turtle(shape='turtle')
bob.color('orange')
# Drawing a hexagon
for i in range(6):
bob.forward(150)
bob.right(60)
turtle.exitonclick()
再次运行您的代码并观看 Bob 画一个六边形。
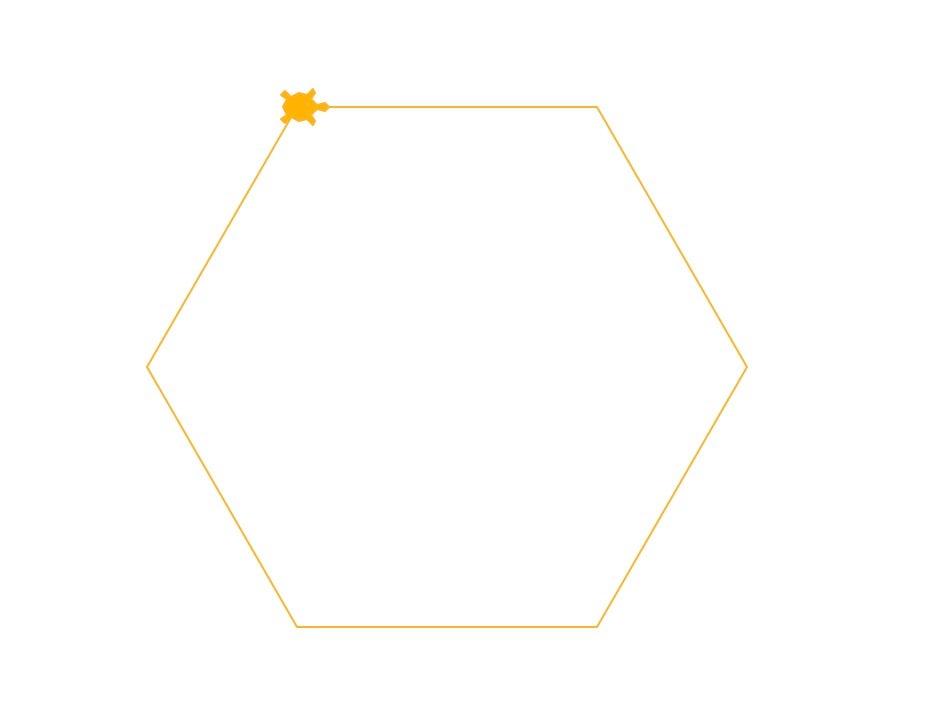
Ayush Sharma 插图,CC BY-SA 4.0
Bob 画一个正方形螺旋
现在尝试画一个正方形螺旋,但这次您可以加快速度。 您可以使用 speed
函数并设置 bob.speed(2000)
,以便 Bob 移动得更快。
import turtle
if __name__ == '__main__':
turtle.title('Hi! I\'m Bob the turtle!')
turtle.setup(width=800, height=800)
bob = turtle.Turtle(shape='turtle')
bob.color('orange')
# Drawing a square spiral
bob.speed(2000)
for i in range(500):
bob.forward(i)
bob.left(91)
turtle.exitonclick()
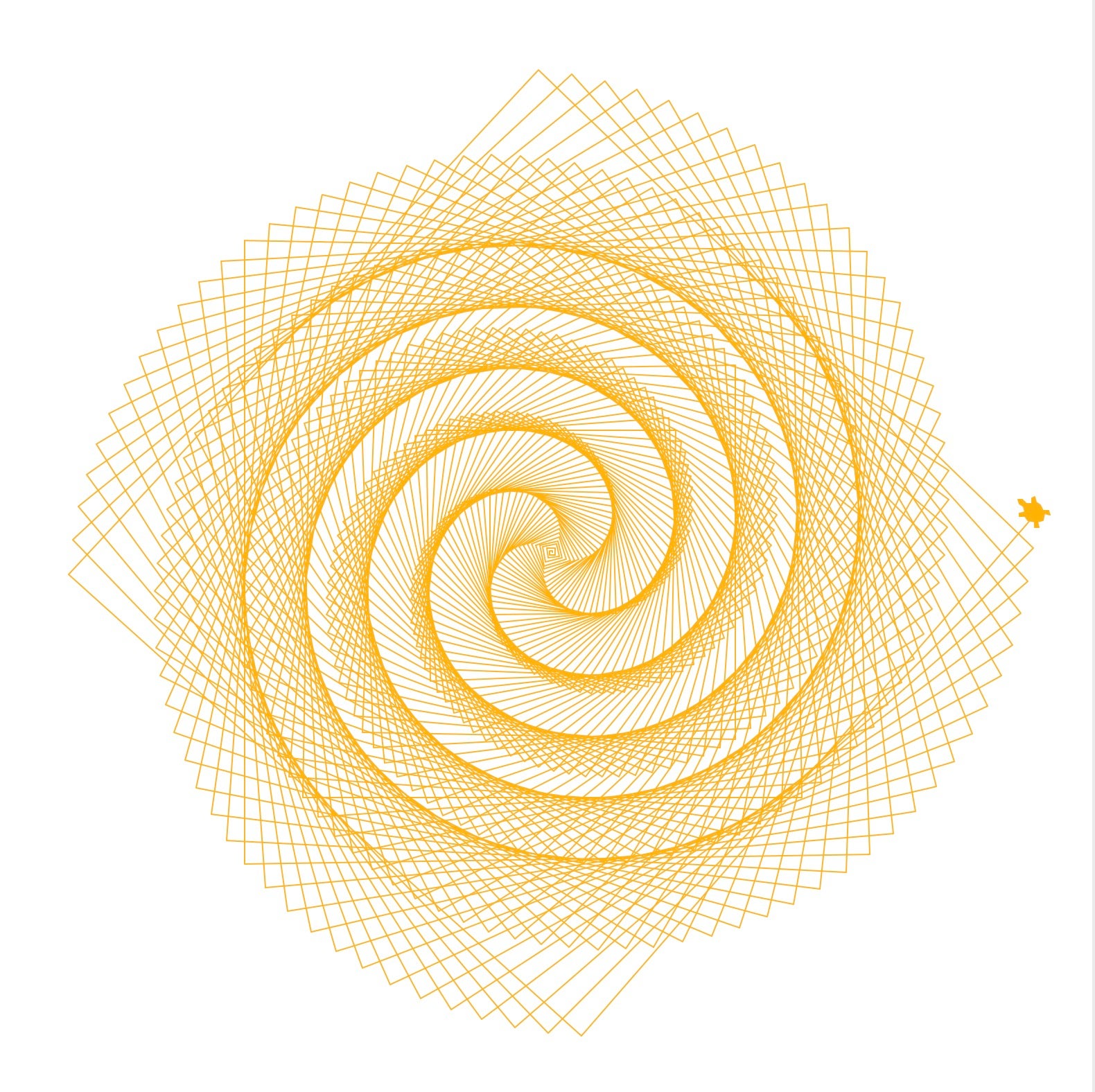
Ayush Sharma 插图,CC BY-SA 4.0
Bob 和 Larry 画一个奇怪的蛇形东西
在上面的例子中,您将 Bob
初始化为 Turtle
类的一个对象。 但您并不限于只有一只乌龟。 在下一个代码块中,创建第二只名为 Larry
的乌龟与 Bob 一起绘制。
penup()
函数使乌龟抬起它们的笔,因此它们在移动时不会画任何东西,而 stamp()
函数会在每次调用时放置一个标记。
import turtle
if __name__ == '__main__':
turtle.title('Hi! We\'re Bob and Larry!')
turtle.setup(width=800, height=800)
bob = turtle.Turtle(shape='turtle')
larry = turtle.Turtle(shape='turtle')
bob.color('orange')
larry.color('purple')
bob.penup()
larry.penup()
bob.goto(-180, 200)
larry.goto(-150, 200)
for i in range(30, -30, -1):
bob.stamp()
larry.stamp()
bob.right(i)
larry.right(i)
bob.forward(20)
larry.forward(20)
turtle.exitonclick()
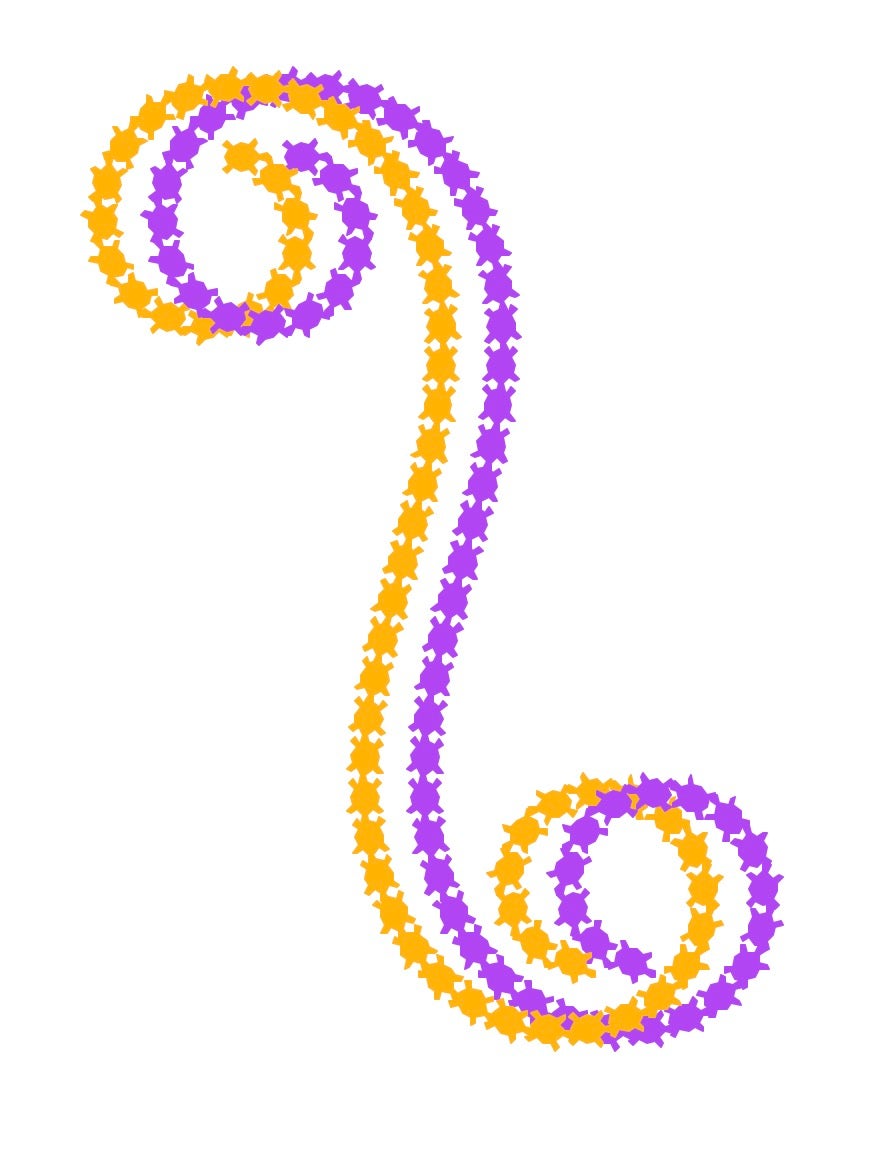
Ayush Sharma 插图,CC BY-SA 4.0
Bob 画一个太阳爆发
Bob 还可以绘制简单的线条并用颜色填充它们。 函数 begin_fill()
和 end_fill()
允许 Bob 用 fillcolor()
设置的颜色填充形状。
import turtle
if __name__ == '__main__':
turtle.title('Hi! I\'m Bob the turtle!')
turtle.setup(width=800, height=800)
bob = turtle.Turtle(shape='turtle')
bob.color('orange')
# Drawing a filled star thingy
bob.speed(2000)
bob.fillcolor('yellow')
bob.pencolor('red')
for i in range(200):
bob.begin_fill()
bob.forward(300 - i)
bob.left(170)
bob.forward(300 - i)
bob.end_fill()
turtle.exitonclick()
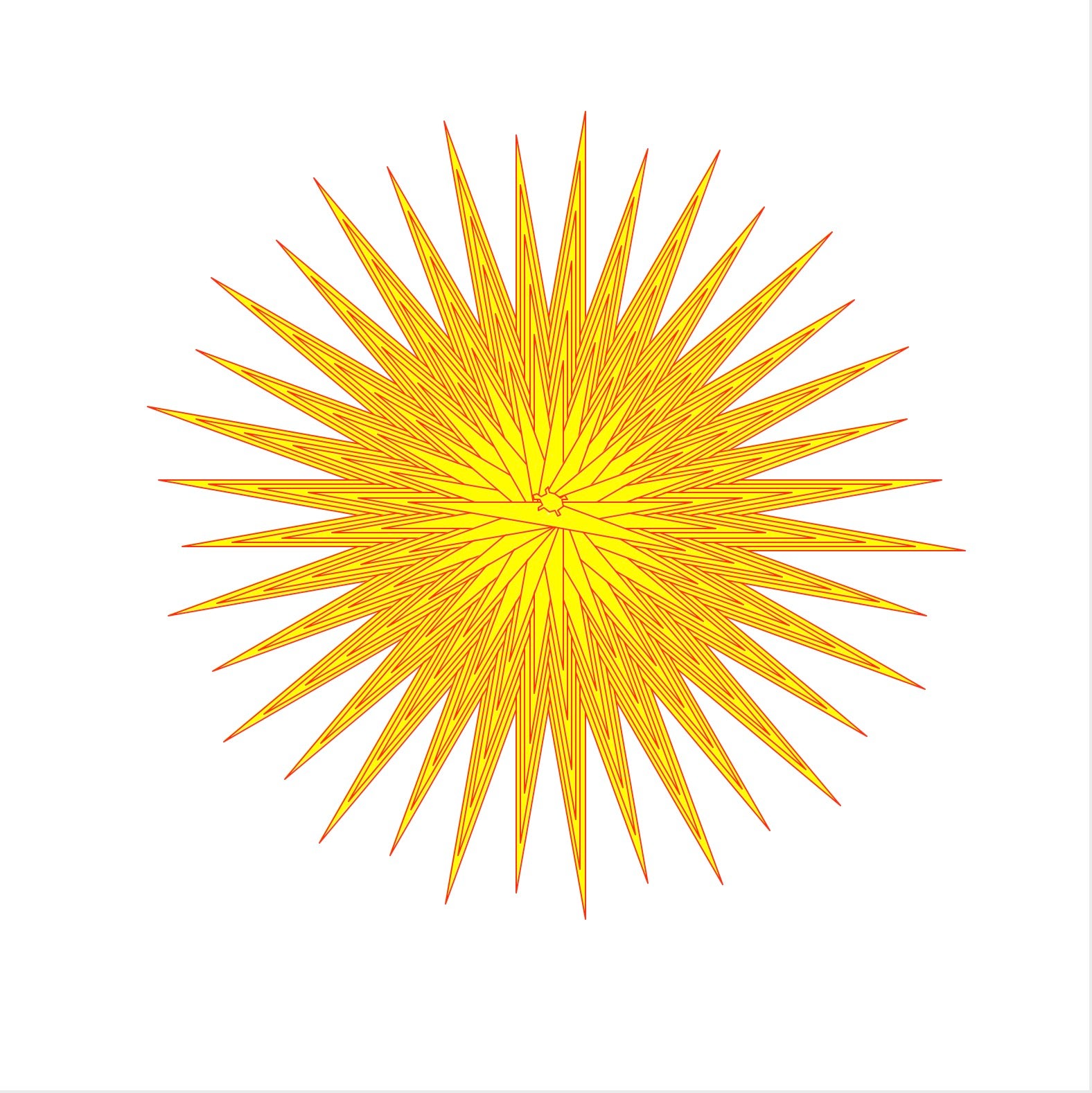
Ayush Sharma 插图,CC BY-SA 4.0
Larry 画一个谢尔宾斯基三角形
Bob 喜欢像下一只乌龟一样用尾巴拿着笔画简单的几何形状,但他最喜欢的是画分形。
其中一种形状是 谢尔宾斯基三角形,这是一个递归细分为较小等边三角形的等边三角形。 它看起来像这样
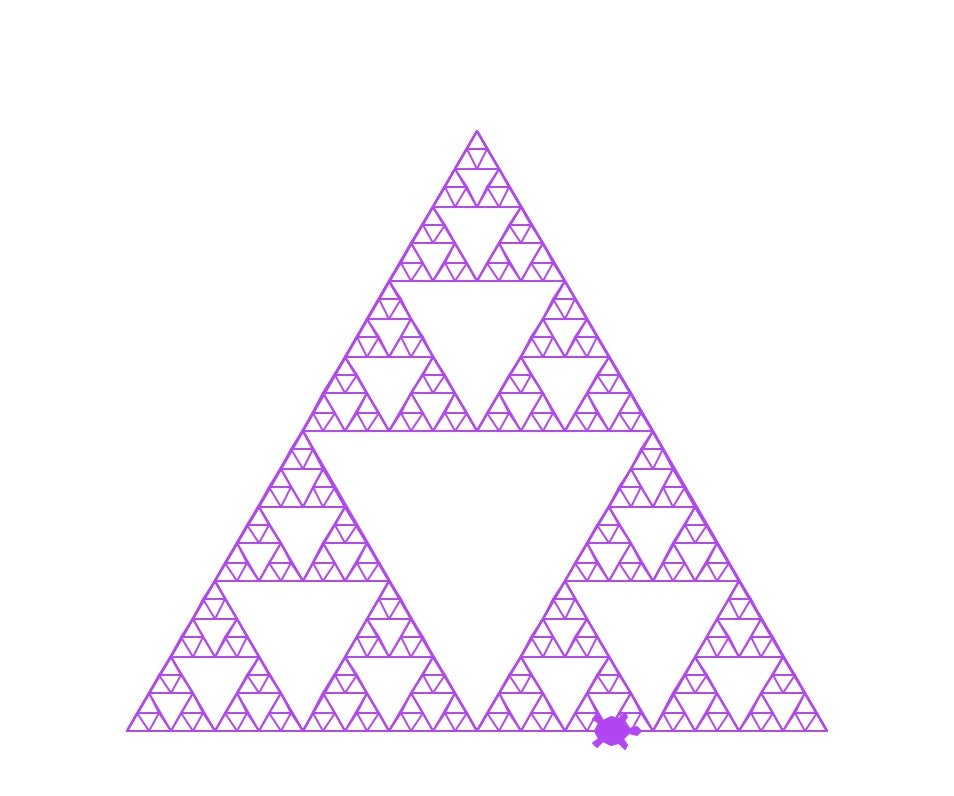
Ayush Sharma 插图,CC BY-SA 4.0
要绘制上面的谢尔宾斯基三角形,Bob 必须更加努力
import turtle
def get_mid_point(point_1: list, point_2: list):
return ((point_1[0] + point_2[0]) / 2, (point_1[1] + point_2[1]) / 2)
def triangle(turtle: turtle, points, depth):
turtle.penup()
turtle.goto(points[0][0], points[0][1])
turtle.pendown()
turtle.goto(points[1][0], points[1][1])
turtle.goto(points[2][0], points[2][1])
turtle.goto(points[0][0], points[0][1])
if depth > 0:
triangle(turtle, [points[0], get_mid_point(points[0], points[1]), get_mid_point(points[0], points[2])], depth-1)
triangle(turtle, [points[1], get_mid_point(points[0], points[1]), get_mid_point(points[1], points[2])], depth-1)
triangle(turtle, [points[2], get_mid_point(points[2], points[1]), get_mid_point(points[0], points[2])], depth-1)
if __name__ == '__main__':
turtle.title('Hi! I\'m Bob the turtle!')
turtle.setup(width=800, height=800)
larry = turtle.Turtle(shape='turtle')
larry.color('purple')
points = [[-175, -125], [0, 175], [175, -125]] # size of triangle
triangle(larry, points, 5)
turtle.exitonclick()
总结
Logo 编程语言是教授基本编程概念的好方法,例如计算机如何执行一组命令。 此外,由于该库现在可以在 Python 中使用,因此它可用于可视化复杂的想法和概念。
我希望 Bob 和 Larry 既有趣又有教育意义。
玩得开心,编码愉快。
本文最初发表在 作者的个人博客 上,并已获得许可进行改编。
评论已关闭。